Functional programming at code clubs
I’ve been mentoring at Brighton Coder Dojo and Code Clubs for a few years now and I’m always on the lookout for new things to try that might broaden the mind, offer alternative approaches and get students thinking differently.
So what about functional programming? How would that work at a code club with 6-16 year olds? Is it even possible in the programming environment we use most often: MIT Scratch?
Functional programming enables you to:
- Write functions that take input and produce output with no side-effects, which makes them easy to reason about and test in isolation, and easy to share across projects
- Use recursion to process lists of things, avoiding the work of loops and loop counters
- Write higher-order functions that take other functions or blocks as input, making it possible to write custom logic or iteration blocks, such as for-each, map, filter or fold.
In addition, functional programming helps you to think differently about algorithms, often resulting in a more concise, elegant solution to a programming challenge. Indeed, many mainstream languages have adopted functional features to bring these benefits to the programmer, see examples in Python and JavaScript.
MIT Scratch is the most popular programming language we use at code clubs and I’ve explored it to see if I can demonstrate functional programming. Unfortunately it’s not possible to create blocks that return values (Reporter blocks – Stack Overflow post) so I need another tool.
Snap! looks like the answer. It’s an extended reimplementation of Scratch that allows you to build your own blocks with many more options than Scratch. In the creator’s words it is Scratch + the power of Scheme.
Modelling a flock of animals
To explore functional programming, we need an interesting app to create, how about Boids? Boids simulate the flocking behaviour of animals, such as birds or sheep. There are 3 simple to state rules:
- separation: steer to avoid crowding local flockmates
- alignment: steer towards the average heading of local flockmates
- cohesion: steer to move toward the average position (centre of mass) of local flockmates
These are simple to state but harder to program! Read on to see some initial versions, with various functional elements. You can click a title to see the code running in Snap.
Sheep version zero
Let’s start with a very simple program: version 0 Here our sheep are moving randomly with no concern for each other. This has one new block (and nothing functional yet): if-on-edge-wrap — and nothing Scratch can’t do:
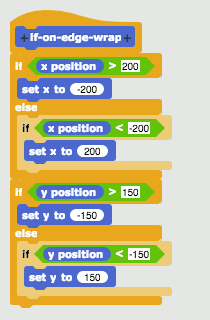
Sheep version 1
Now for version 1 Here our sheep are moving randomly, but they know when they are near another sheep and flash. I’ve created a new control block for-each so that we can run the set of instructions on every sheep.
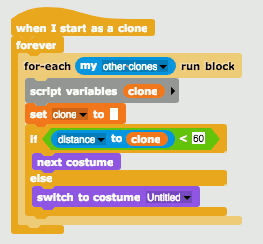
The first two lines in the block create a local variable for each of the other sheep. We check the distance from us to this sheep and switch costume if it’s less than 60. The new for-each block makes it easy to do things to every element of the list, without having to do the usual loop work in Scratch.
Here’s the definition of the block, it takes a list and a code-block as parameters and uses recursion to work through the items of the list apply the block of instructions (above) to each. You can see the exit clause in the empty if-statement, if there’s no list left we’re all done.
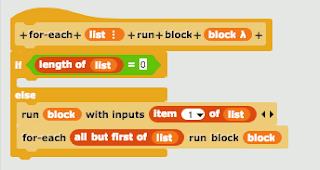
Sheep version 2
Version 2 - Now our sheep like the mouse pointer and move towards it. There are several new functions to calculate the force applied to each sheep from the mouse.
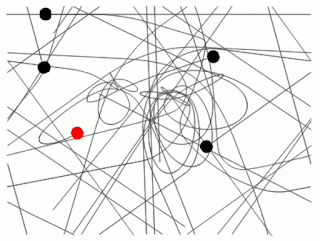
Here’s the main block of code that runs for each sheep:
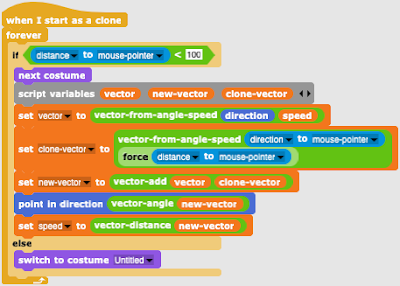
You can see them at the bottom of the left hand panel in the Operators section in Snap.
To do…
There’s lots left to explore, for example:
- The sheep move too fast, maybe they should have a maximum speed?
- While we’ve shown how the sheep react to the mouse pointer, they don’t react to each other yet; Version 1 has a starting point for this behaviour in that they know when they are close together.